Froggy Game
Evolving from the Classic: Design and Architecture
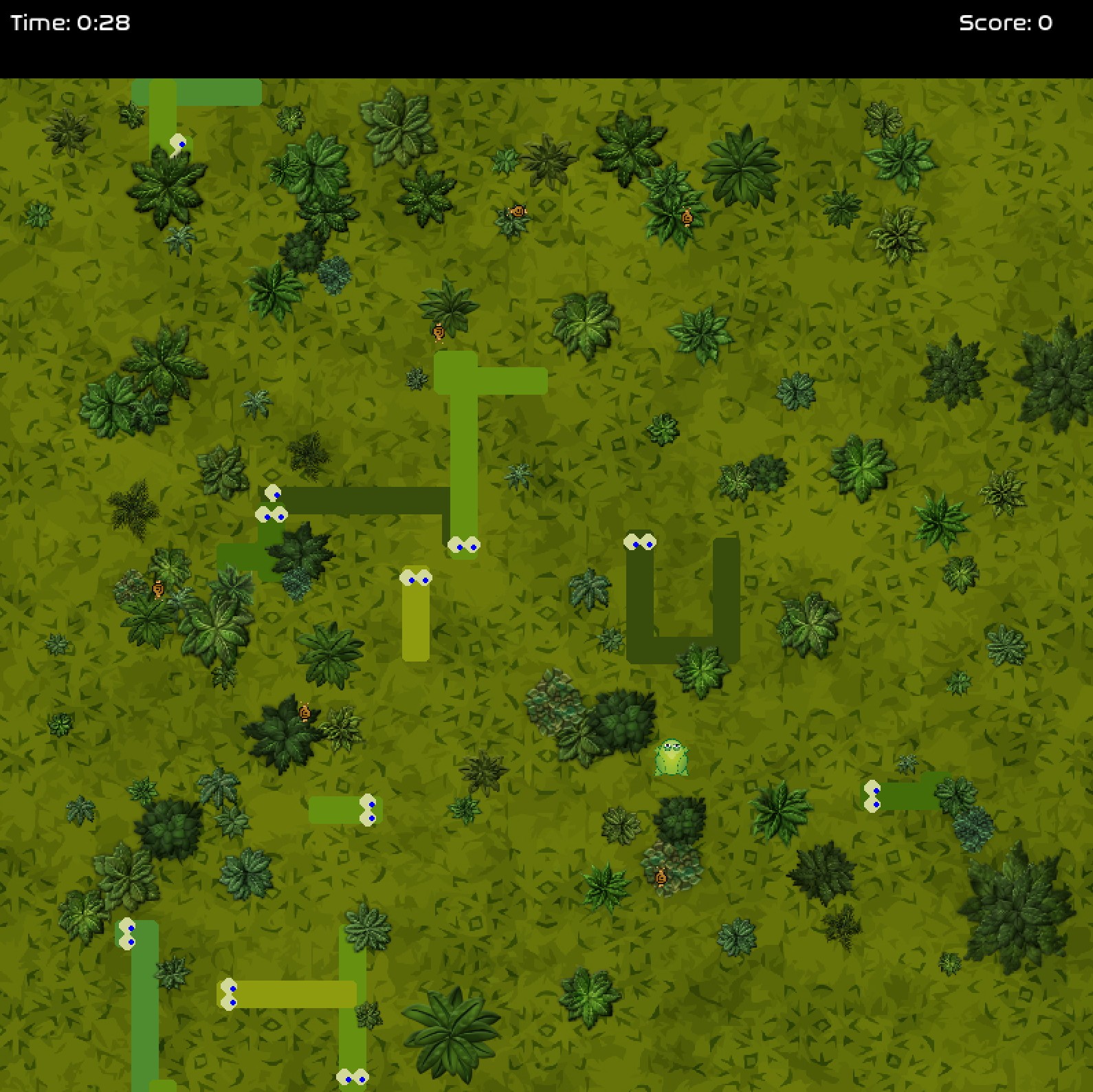
Overview
In this project, I make a Froggy Game with the goal of delivering an exciting and fun gaming experience while also making sure the codebase remains modular and easy to maintain. The game is inspired by the Crossy Game and Frogger, with the old-school feel combined with new improvements. One of the coolest features is the revised snake design, which draws from my prior Snake Game but with improved graphics and a smoother frame rate. This helps the gameplay feel more fluid and enjoyable.
The architecture of the game is built around the separation of concerns and making sure each part of the game is reusable and clear. This means each class has its specific responsibility in the game such as managing the frog's movements or handling snakes, which keep everything organized. In such a way, this makes the game much easier to be updated and maintained later on. It's something like building with Lego blocks: each part fits together, and if I want to add something or correct something, I don't have to tear it all down and be confused when I look at my previous Snake Game.
To have the code flexible and growable but not become a mess, I followed the design principles according to the Gang of Four design patterns. These patterns help the game stay robust and adaptable so that future changes or additions to the game can be made without breaking the existing code. It's all about building a solid foundation now, so the game can continue to evolve in the future whether it's adding new challenges, better graphics, or even expanding the gameplay mechanics.
View JavaScript Shortened VersionMy Role
- Game Illustrator
- Developer and Programmer
Language
- Python
Personality
- Fun
- Intuitive Control
- Engaging
Froggy Game Class Diagram
I divide the game’s functionality into three main categories: Main Application, Game Components, and Scenes, supported by Utilities and Effects. These categories group related classes together, following the principle of cohesion, where each class and module has a well defined purpose. This structure makes sure that future me can easily locate and modify code relevant to a specific feature or functionality.
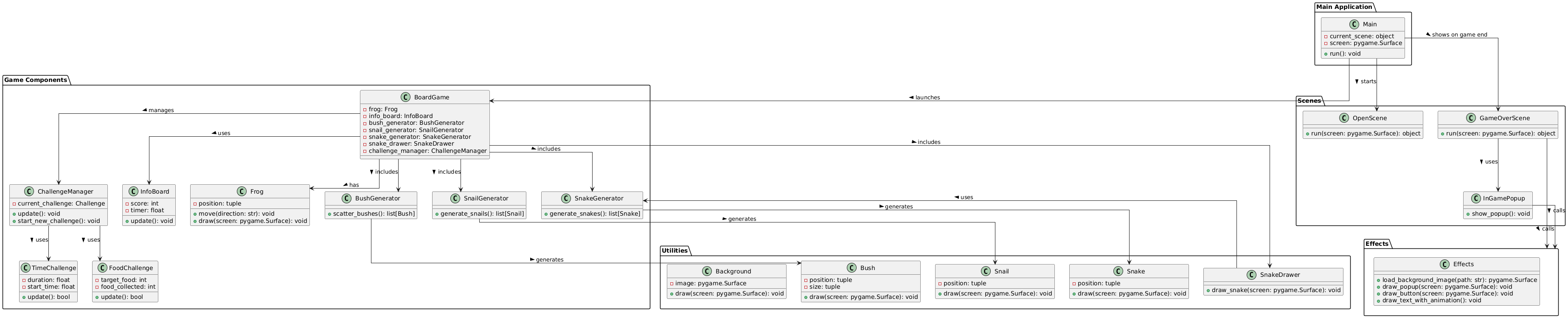
Main Object Diagram
The Main class acts as the entry point of the application. It initializes the game, manages the transitions between scenes (like the opening menu and game over screen), and keeps the gameplay flow organized. The scene-based design follows the State Pattern, as the game changes its behavior depending on the current state or scene (e.g., OpenScene, BoardGame, GameOverScene).
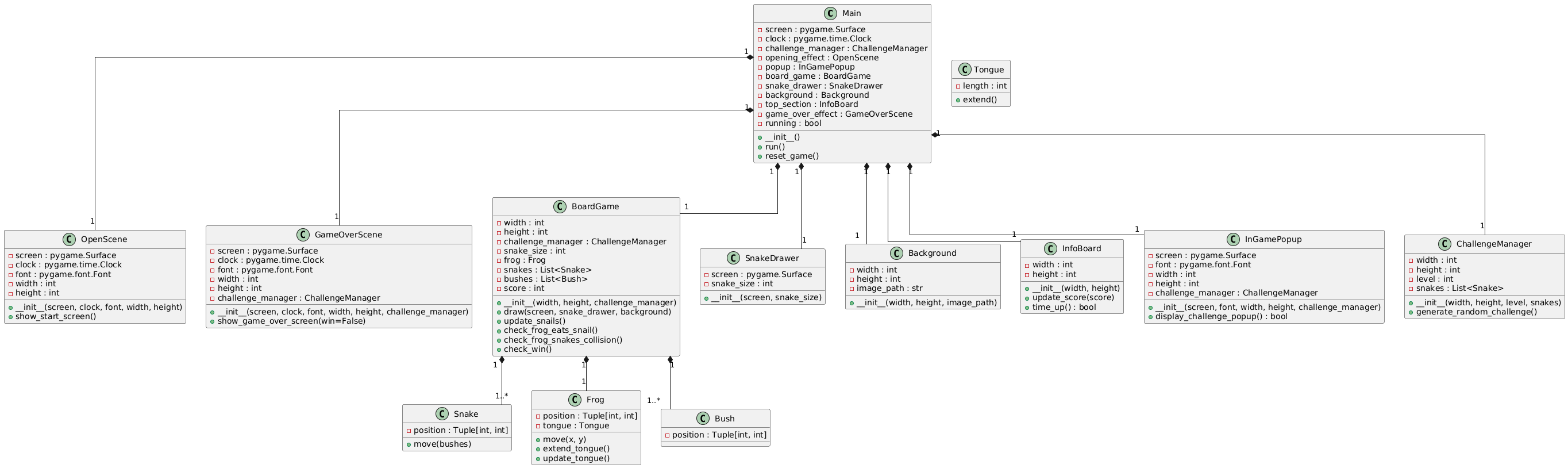
BoardGame Object Diagram
The Frog starts in the middle of the bottom part of the board game. Snails are generated on top of bushes, and I designed them so that they don’t leave the bush. The Snakes are generated randomly with various shades of green, length and speed. They can’t go through big bushes, so they have to detour. To avoid snakes getting stuck, bushes are calculated so that when they are generated, the gap between different groups of bushes are at least the snake size.
The core mechanics of the game are wrapped in the BoardGame class. This class acts as the heart of the gameplay, combining the frog, challenges, and game objects together. BoardGame manages the interactions between components, including the placement of bushes, the movement of the frog, and the appearance of snails. Each of these components is controlled by a class, making it easier to add new mechanics or adjust existing ones.
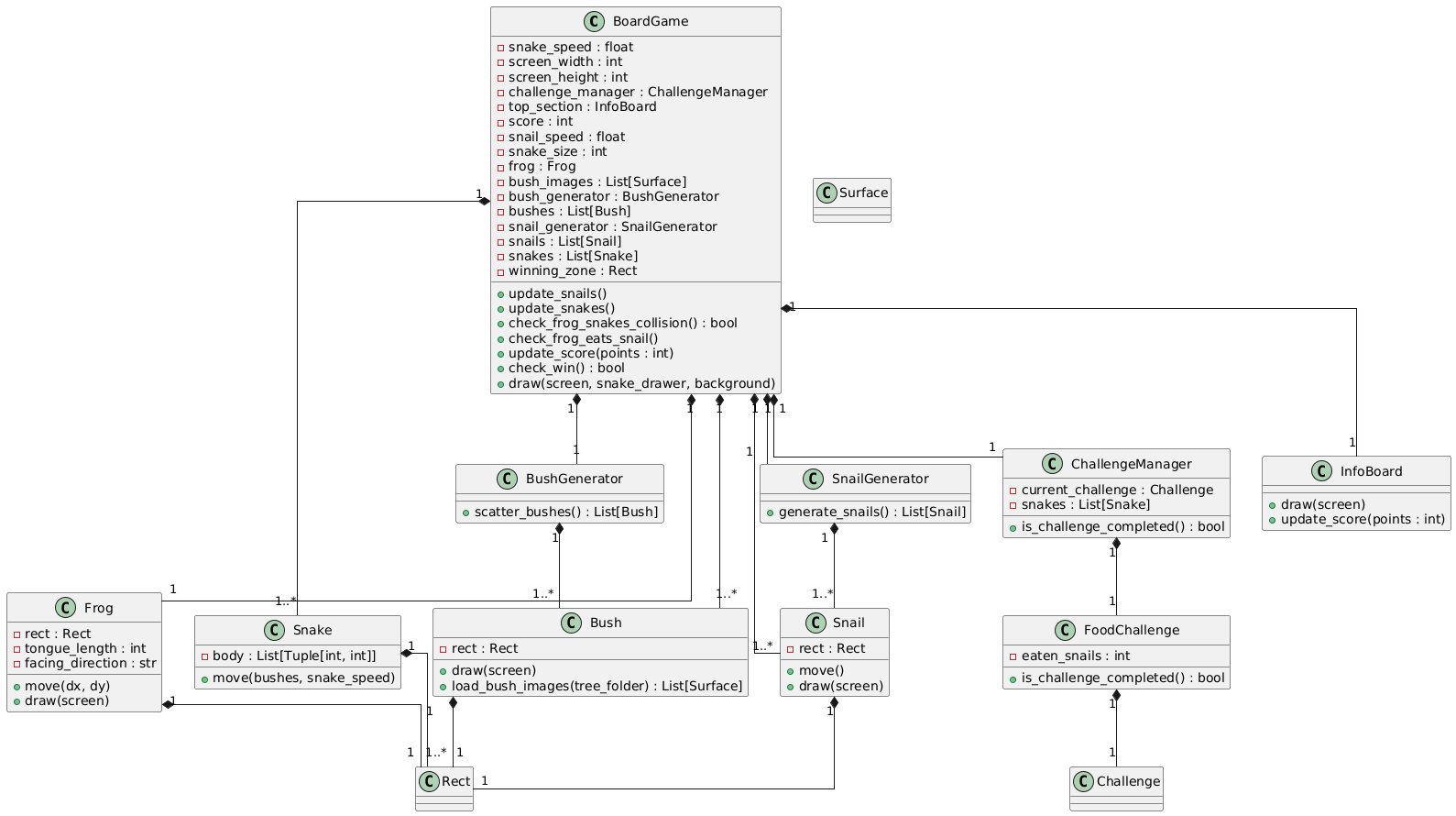
Frog Object Diagram
The Frog class is modular, wrapping behaviors like movement, sprite management, and tongue animations. It uses patterns like Factory Method for sprite loading, State Pattern for tongue animation, and Strategy for dynamic sprite updates. Encapsulation makes sure a clear interface, while sticking to the Single Responsibility and Open-Closed principles. Its design is scalable and reusable, supporting future extensions like new actions or behaviors without modifying existing functionality, ensuring maintainability and adaptability.
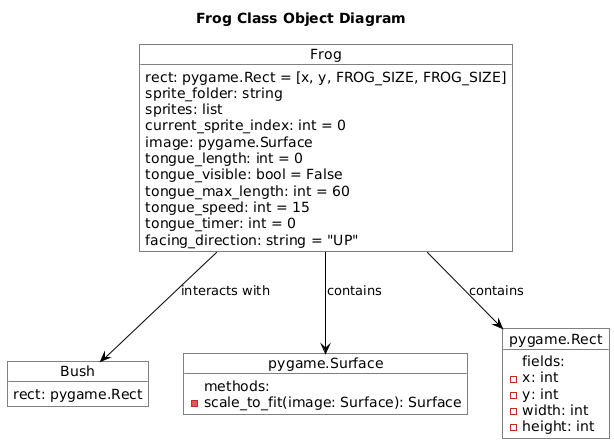
Bush Object Diagram
The Bush class is encapsulated in maintaining its position, size, and appearance, while the static method for loading images applies the Factory Method Pattern, centralizing bush image creation. BushGenerator introduces a strategy for scattering bushes. This creates proper placement through validation. This design sticks to the Single Responsibility Principle by separating bush behavior from generation logic, making the system modular, reusable, and adaptable to changes like new placement rules or bush types.
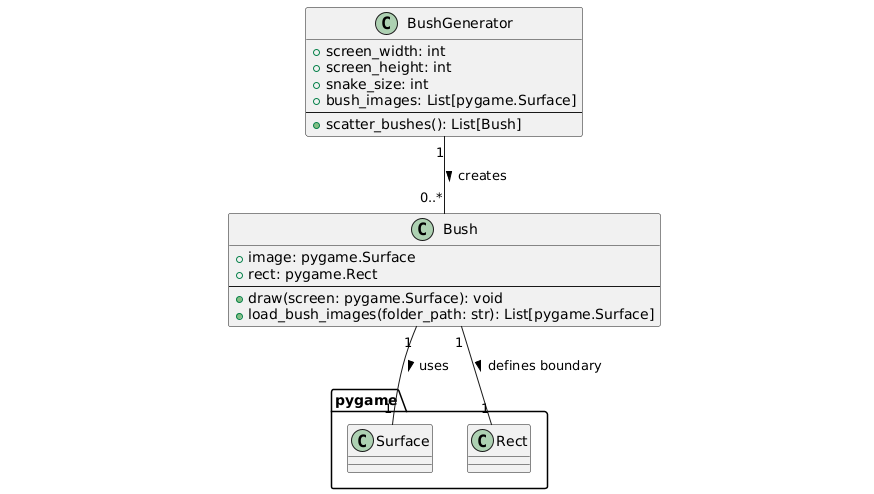
Snail Object Diagram
Similarly, the Snail class demonstrates encapsulation by managing a snail's appearance, movement, and animation within a single unit. It applies the State Pattern to manage direction changes and sprite updates based on time, ensuring smooth animations. The SnailGenerator separates the logic for placing snails on bushes, adhering to the Single Responsibility Principle. By centralizing generation, it uses a Factory Method-like approach to create snails dynamically, ensuring modularity. This design enables flexible customization and cleanly integrates with the game's bush-based environment.
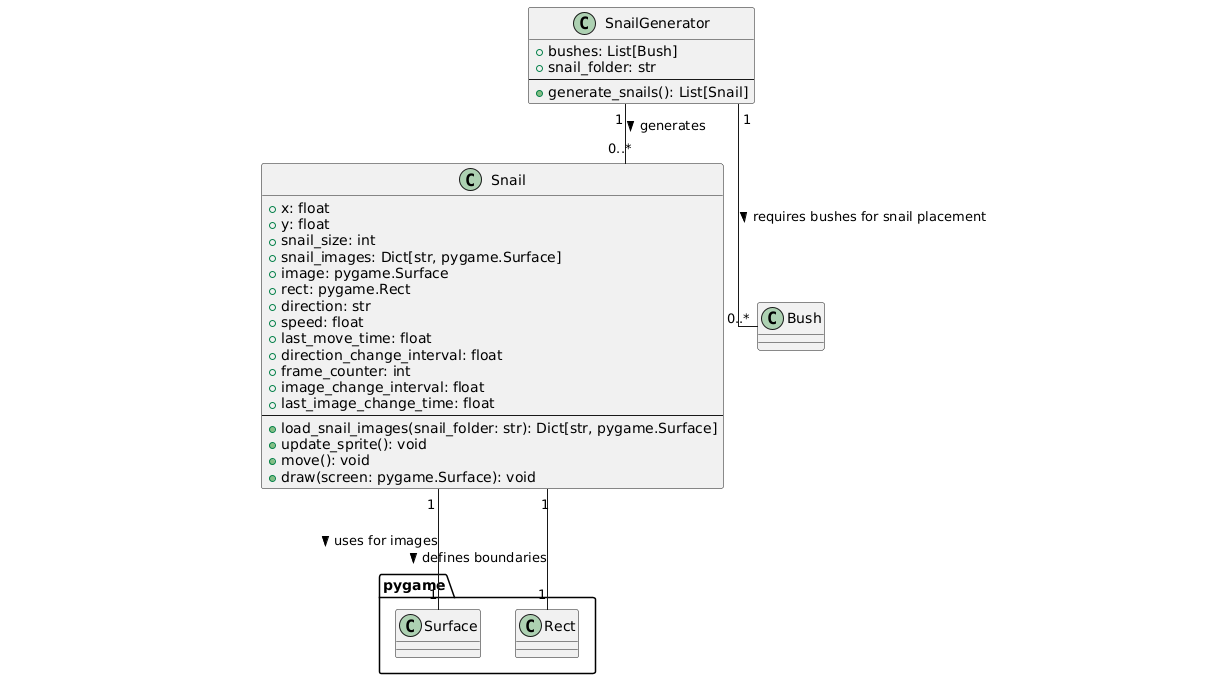
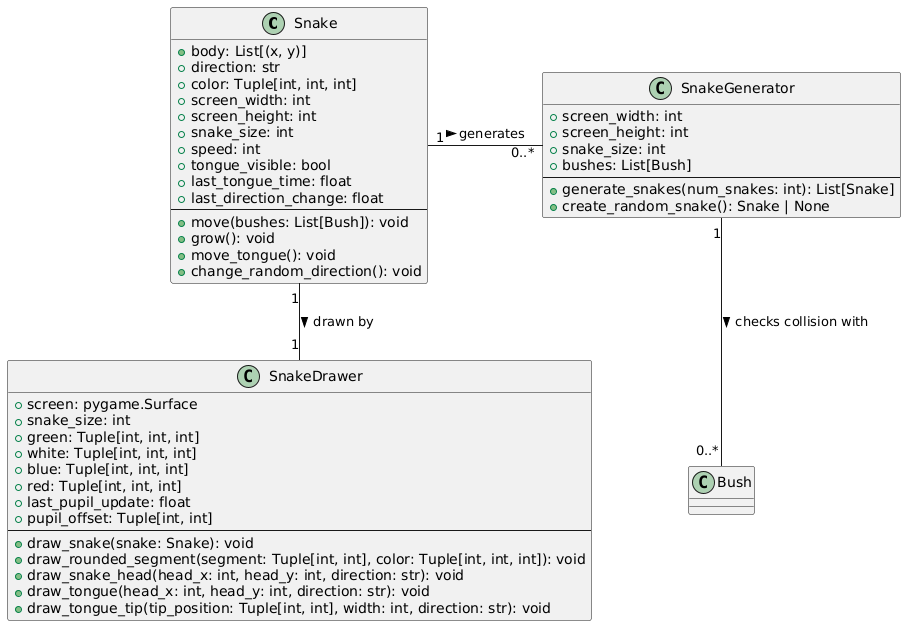
ChallengeManager Object Diagram
The ChallengeManager introduces challenges like TimeChallenge and FoodChallenge to diversify gameplay. Each challenge class has specific logic, promoting adherence to the Single Responsibility Principle. This separation of concerns ensures that updates to one type of challenge will not affect others.
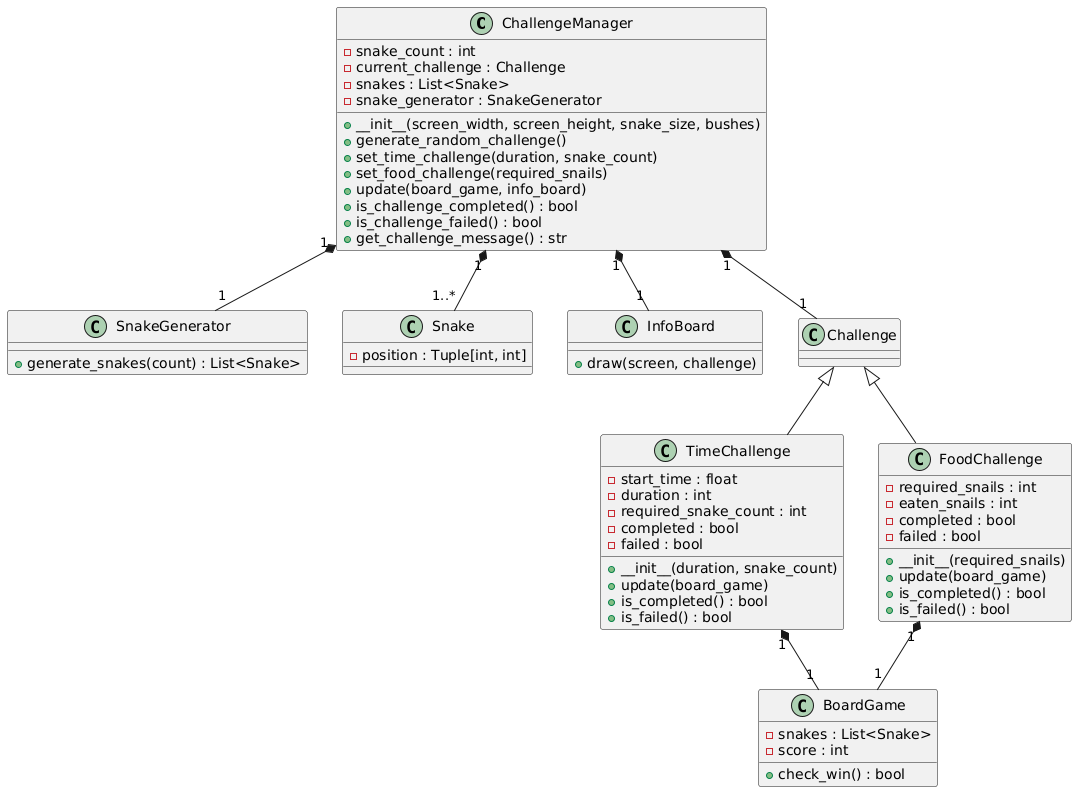
Scenes
The scene-based architecture simplifies transitions and focuses on specific game states. The OpenScene displays the game's opening screen with animations, while the GameOverScene manages the behavior after the player loses. These classes use helper functions from the Effects module for common visual elements like popups and animations, ensuring reusability and reducing redundancy.
The use of scenes follows the Strategy Pattern because the behavior of the game changes based on the active scene. This modularity allows future me to add new scenes, such as a settings menu or a pause screen, without altering the core game logic.
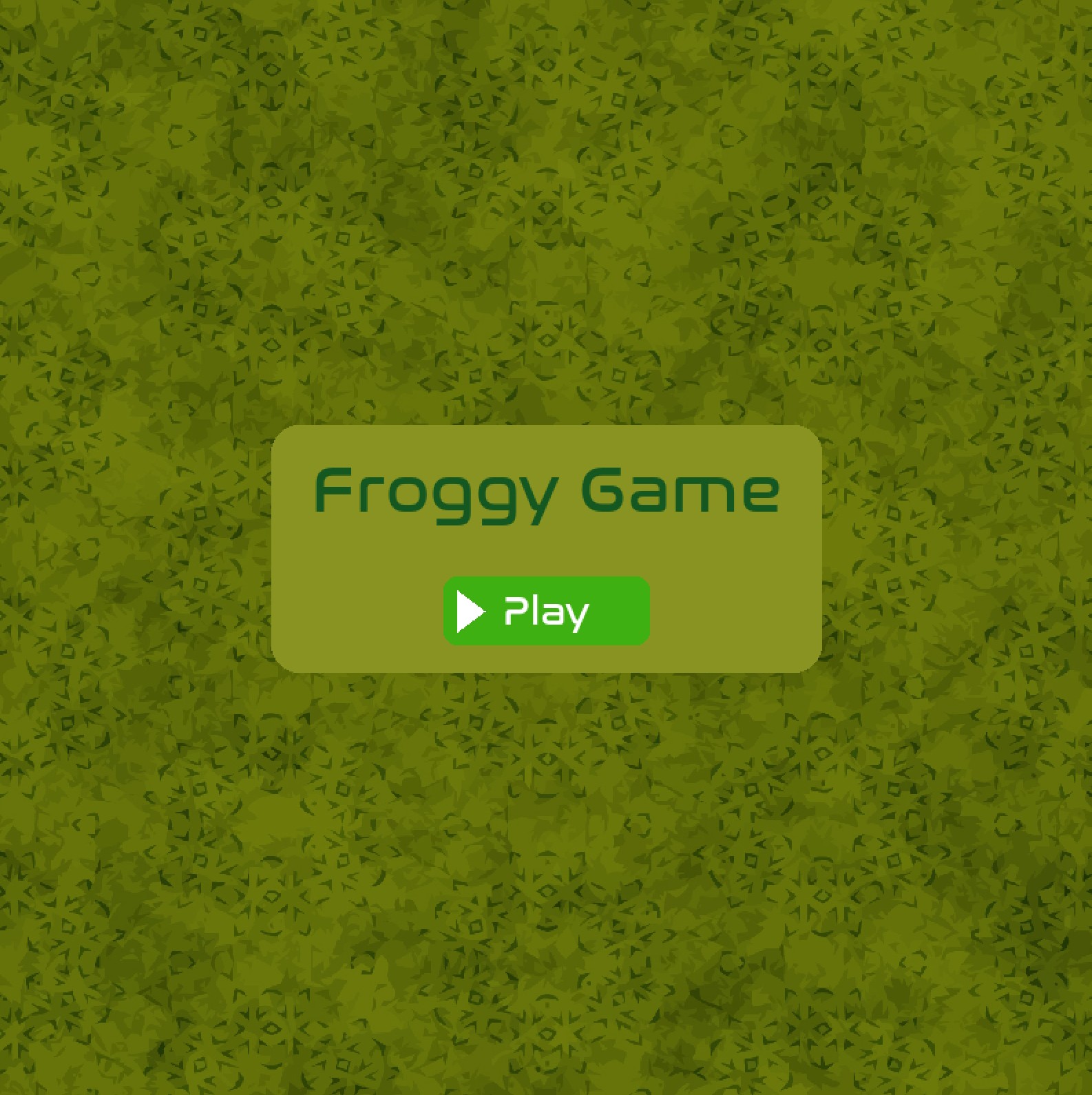
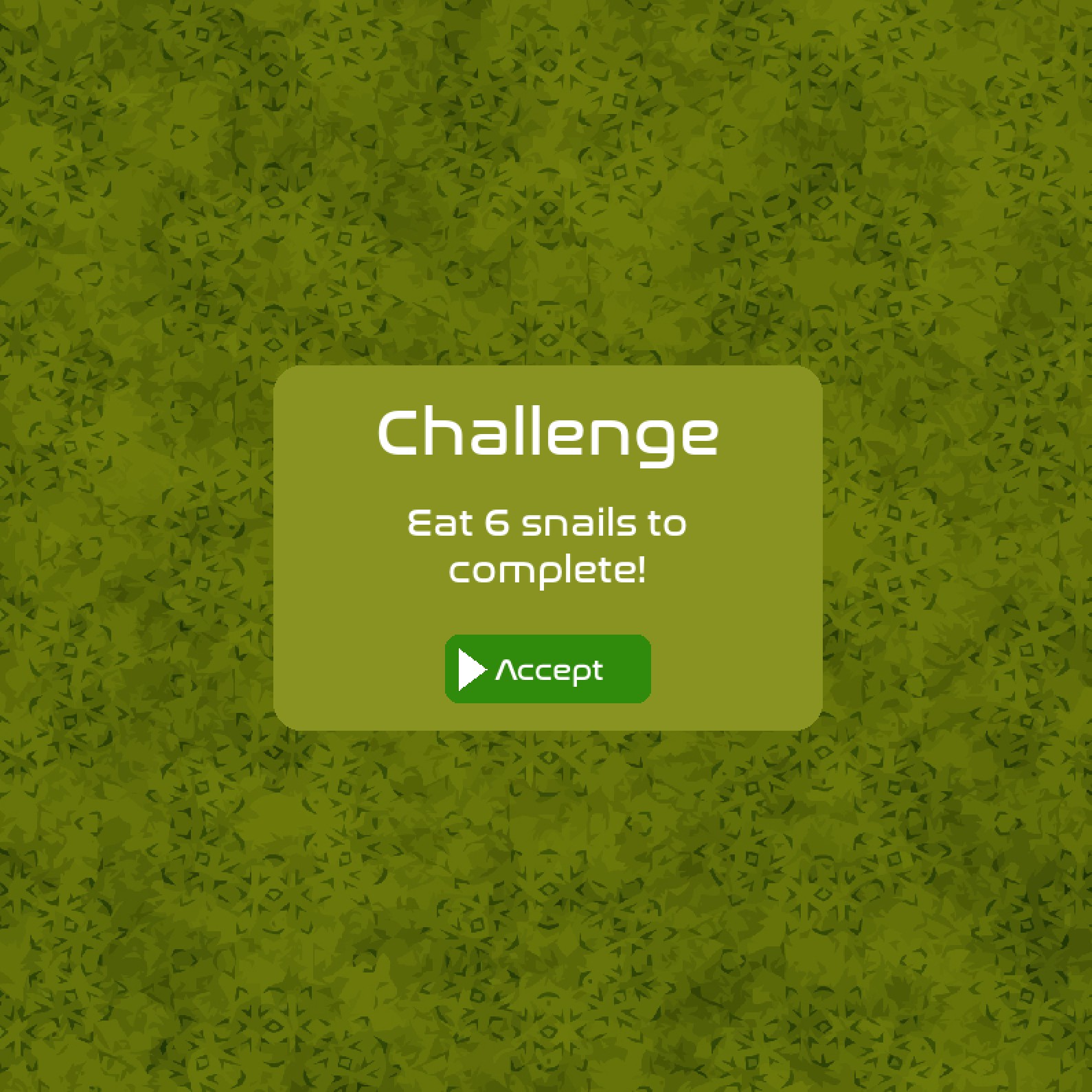
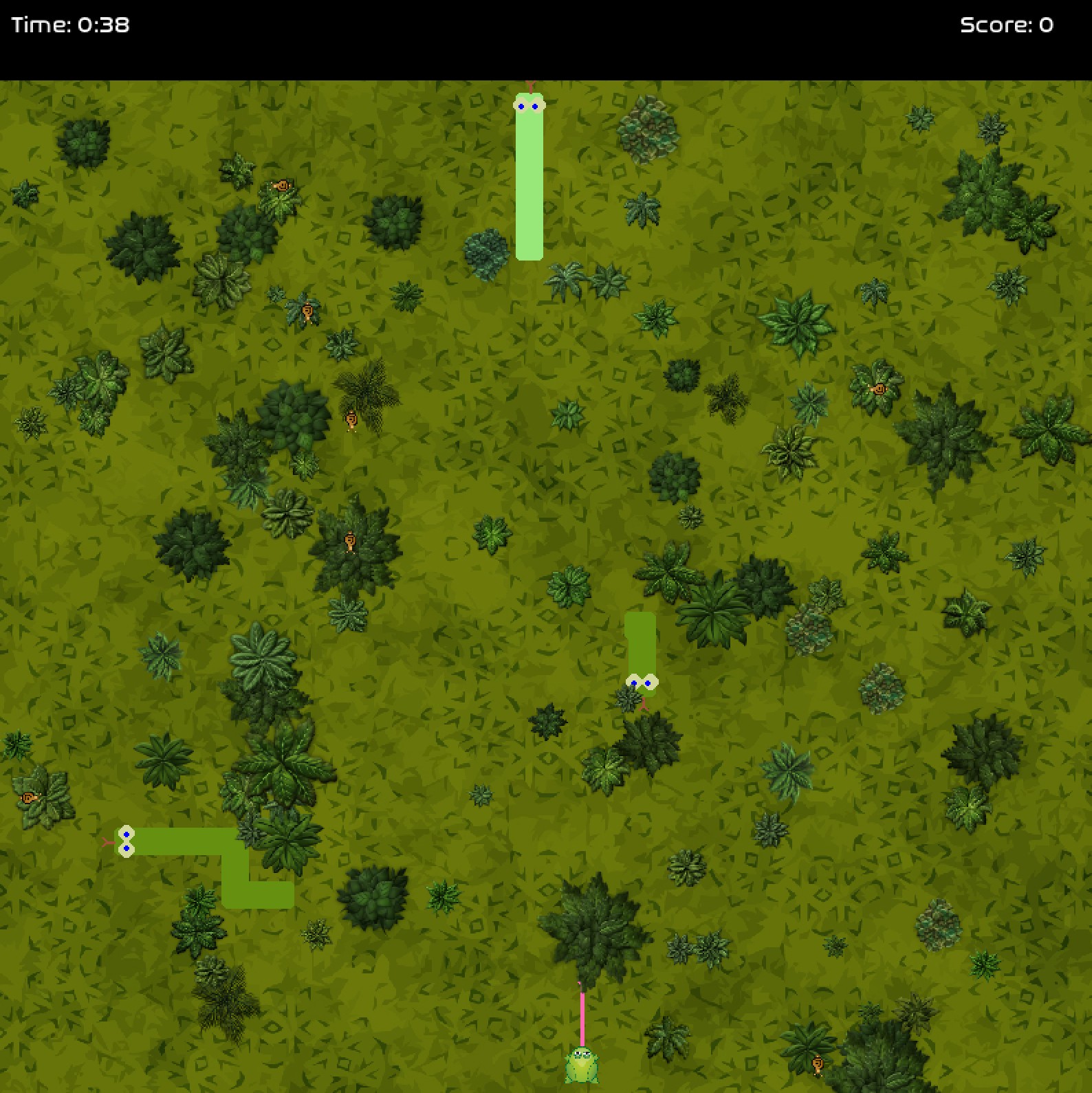
Utilities and Effects
The Background, SnakeDrawer, and InfoBoard classes handle auxiliary tasks such as drawing backgrounds, managing snake visuals, and displaying player information. These components are isolated to prevent clutter in the main game logic. The Effects module centralizes common visual tasks like loading images, creating animations, and drawing buttons. This organization ensures that visual changes are made in one place, following the Facade Pattern by providing a simplified interface for complex rendering operations.